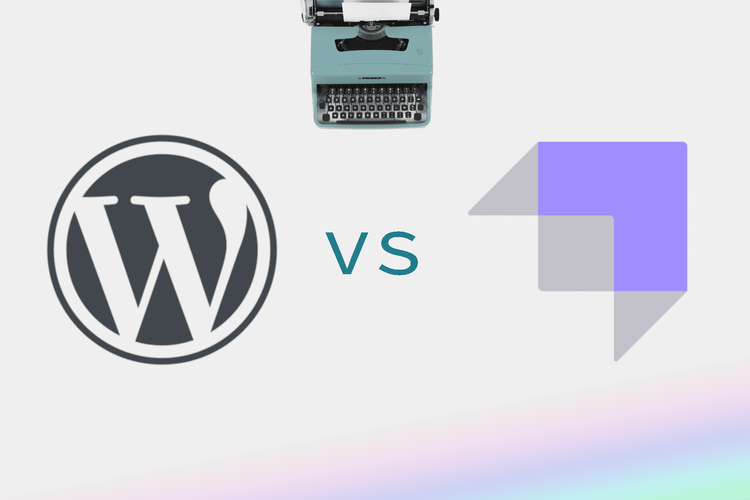
Strapi or Headless Wordpress?
CMS (content management systems) are used for a wide range of applications: from personal blogs and landing pages to E-commerce and B2B software. As of Jamstack Community Survey, WordPress is being used as a headless CMS by 21% of websites. Strapi is being used by 17%. So, Strapi vs WordPress: which one to use when?
According to Jamstack Community Survey top 5 most popular CMS are WordPress, Contentful, Strapi and Drupal.
In short
If you don't have any prior technical experience (and don't want to know why "2" + "2" = "22"
), use WordPress. It already has an admin panel and a frontend (a website) for your users. It's very easy to upload a theme or add more features with ready-made plugins (like a carousel or a form builder). A cherry on top is that there are a lot of hosting providers which will set up and configure a server for your website.
An API can also be used to make a custom frontend, making WordPress headless.
Strapi, being a headless CMS, gives full control over how a website looks. It operates on an idea of a "Collection type". A collection type is like a form: it has a set of fields to fill in and content that fills in those fields. Fields can be constrained, such as "a text field can be no longer than 500 symbols", "a field is required", etc. Fields can also contain one-to-one or one-to-many relations.
Strapi supports server side plugins, like ezforms. They allow extending Strapi's API, but they do not have any UI elements for the website visitors.
With that in mind, Strapi can be used as a ready-made backend for many more things than a blog, like an online store or a classifieds website.
API comparison
In this article we'll compare only APIs for fetching content, as user management is sometimes done using third party providers.
WordPress has a JS client to interact with its API.
It should be activated server side:
wp_enqueue_script( 'wp-api' );
And then it can be used on the client. WP API features an object model pattern where you first create an empty object, then populate it with data:
// create a new post
var post = new wp.api.models.Post( { id: 1 } );
// fetch data for post with id = 1
post.fetch();
You can also get object's relational data:
// Get a collection of the post's categories (returns a promise)
// Uses _embedded data if available, in which case promise resolves immediately.
post.getCategories().done( function( postCategories ) {
// ... do something with the categories.
console.log( postCategories[0].name );
} );
Strapi's REST API exposes content via API endpoints.
There are JavaScript/Typescript packages for interacting with Strapi API, such as @iamsamwen/strapi-api. This package provides "a wrapper of strapi api calls util class to simplify the code to manipulate strapi data and media files".
This leaves a developer with a choice which design patterns and project structure to use.
Strapi's API allows to query any Collection type:
// GET http://localhost:1337/api/restaurants
{
"data": {
"id": 1,
"attributes": {
"title": "Debra's Burgers",
"description": "Fresh beef and shrimp burgers"
},
"meta": {
"availableLocales": []
}
},
"meta": {}
}
By default, Strapi does not fill in object relations and media attachments. Unlike WordPress, you can fetch those in one call. populate=*
parameter allows populating all object's relations and media fields.
// GET http://localhost:1337/api/restaurants?populate=%2A
{
"data": {
"id": 1,
"attributes": {
"title": "Debra's Burgers",
"description": "Fresh beef and shrimp burgers",
// headerImage is not shown without "populate" parameter
"headerImage": {
"data": {
"id": 1,
"attributes": {
"name": "17520.jpg",
"alternativeText": "Debra's Burgers building",
"formats": {
// ...
}
// ...
}
}
}
},
"meta": {
"availableLocales": []
}
},
"meta": {}
}
Specific parameters can also be populated, reducing the response size.
Note: both WordPress and Strapi API support GraphQL, but neither support it out of the box, and it is not covered in this article. GraphQL + WordPress, GraphQL + Strapi
(Admin) user management
Managing website's admin users is important if you're not the only one writing a blog.
WordPress supports 5 user roles: Administrator, Editor, Author, Contributor, and Subscriber.
Administrator has access to all the administration features within a single site.
Editor can publish and manage posts, including the posts of other users.
Author, can only publish and manage their own posts.
Contributor can write and manage their own posts, but cannot publish them.
Subscriber is somebody who can only manage their profile. This role can be used for subscription based websites.
Existing roles can be modified and more can be added only using with a WordPress plugin.
The free version of Strapi has only 3 roles: Author, Editor and Super Admin. As Strapi is using Collection types, role based access can be configured for each of the fields in a collection. Free version of Strapi also allows specifying which locale which the user can edit.
Paid version of Strapi allows adding more admin user roles, configuring create, read, update, delete field access and defining access conditions, such as "only a user who created this object can modify its fields".
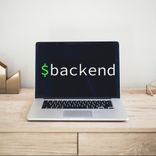